When we run an Angular application and serve it on a port number say ( 4200 ), we get our application running at http://localhost:4200
This is the only path our browser understands, and hence it can’t serve different destinations on different paths such as http://localhost:4200/items. But, What if we have multiple paths in the same application ?
After this, you can define an array of your paths and destination pages.
This is the only path our browser understands, and hence it can’t serve different destinations on different paths such as http://localhost:4200/items. But, What if we have multiple paths in the same application ?
How to achieve custom URL paths
Javascript Router: It makes our lives easier by changing the destination whenever the path changes. In technical terms, by changing the application state whenever the browser URL changes and vice-versa.
These Routers are written in certain Javascript Frameworks and for Angular we have it packaged as
@angular/router
The
@angular/router
contains the shown terms: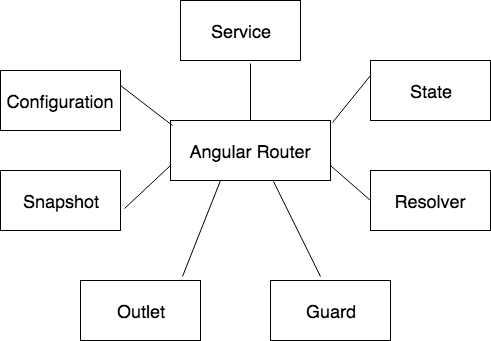
- Service: This is a global service present inside the Angular Application.
- State: The state of Router is maintained by Router State.
- Configuration: It maintains information about all the routing states which is present inside the application.
- Guard: This is a code that runs whenever the router is activated, deactivated or loaded.
- Snapshot: It will provide access to state and data for a particular route node.
- Resolver: The script that will fetch data before the requested page is initiated.
- Outlet: This is the location in DOM for router outlet to place it’s components.
Now Let's Code:
To tell the paths and destinations to your browser, you need to import the
RouterModule
and Routes from @angular/router
into your app.module.ts
file.
> import {RouterModule, Routes} from '@angular/router';
const routes: Routes = [ { path: '', redirectTo: 'items', pathMatch: 'full' }, { path: 'items', component: AppComponent } ];
Note: Here, the pathMatch is specifying a strict matching of path to reach the destination page.Now, you need to pass the routes array in the
RouterModule
using RouterModule.forRoot(routes)
and have to add it to imports
> RouterModule.forRoot(routes)
app.module.ts
file will look like this:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import {RouterModule, Routes} from '@angular/router'; import { AppComponent } from './app.component'; const routes: Routes = [ { path: '', redirectTo: 'items', pathMatch: 'full' }, { path: 'items', component: AppComponent } ]; @NgModule({ declarations: [ AppComponent, ], imports: [ BrowserModule, RouterModule.forRoot(routes) ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }